How to Set the Creator Metadata for a PDF With iText7 and C#
In this post I'll take you through setting the creator metadata for a PDF with iText7 and C# in a way you can understand what's happening and just copy it into your codebase.
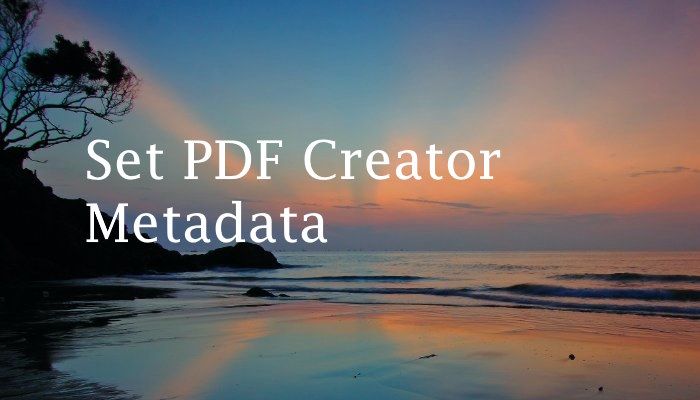
The following is a plug and play block of code that makes no assumptions on your code base. There will most probably be a more efficient way to incorporate this for your needs such as passing more things around by reference, or having guard clauses. This example also uses the newer C#8 using
declaration syntax, but feel free to swap that out with the usual using
statement syntax.
This code below takes in a byte[]
of the PDF, safely opens it and sets the creator metadata field. If you already have the PdfDocument
object in your code, then your task is even easier.
For this example, we use both using
styles as once we've modified the PDF we need to close the PdfDocument
object in order for changes to be observed in the output Stream
.
public byte[] SetCreatorMetadata(byte[] pdf, string creator)
{
using var inputStream = new MemoryStream(pdf);
using var reader = new PdfReader(inputStream);
using var outputStream = new MemoryStream();
using var writer = new PdfWriter(outputStream);
using (var document = new PdfDocument(reader, writer))
{
var documentInfo = document.GetDocumentInfo();
documentInfo.SetCreator(creator);
}
// The PdfDocument must be closed first to write to the output stream
return outputStream.ToArray();
}
Want to quickly spin this up to play with it? You can get your PDF byte[]
via:
var pdf = File.ReadAllBytes(@"C:\document.pdf");