The Weird Things Roslyn Gets up To
Ever wanted to see some strange, interesting, and weird .NET behaviour and features? What about bizarre C# to baffle? If so, then welcome to this little curated and peculiar collection.
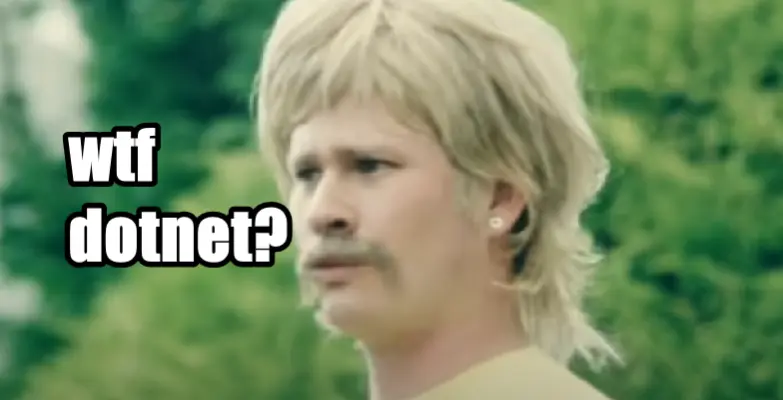
We've all seen the JavaScript examples where NaN === NaN
is false
etc. If not, check out some examples in the repo below:
Some you might've seen them, some might be new to you! But what Strange things can we dig up in .NET/C#? Here's a collection I've gathered over the course of 2022.
Smallest Valid C#
At least at the time of .NET 6:
{}
You can see for yourself in this Sharplab.io snippet.
Originally this came to me as a tweet from @Nick_Craver:
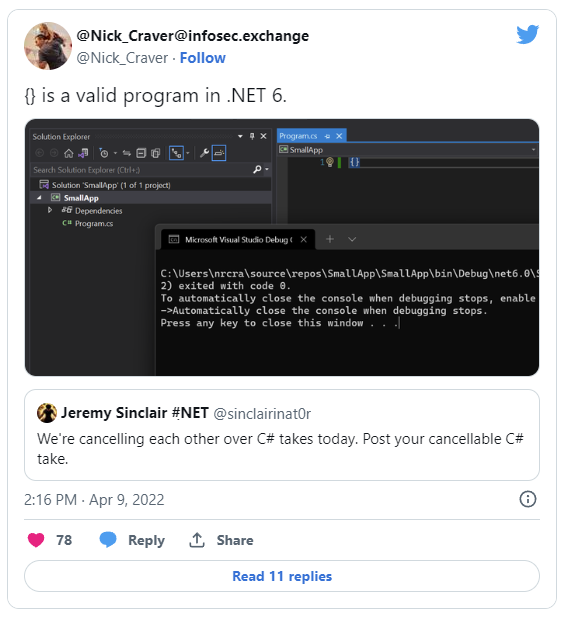
If you want to dig into it, there is a really fun post from @nietras1 exploring step by step what the smallest valid C# is.
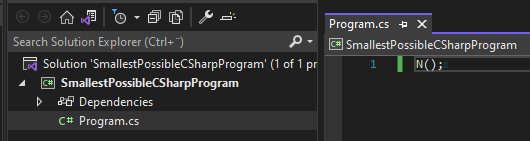
It's All Greek to Me
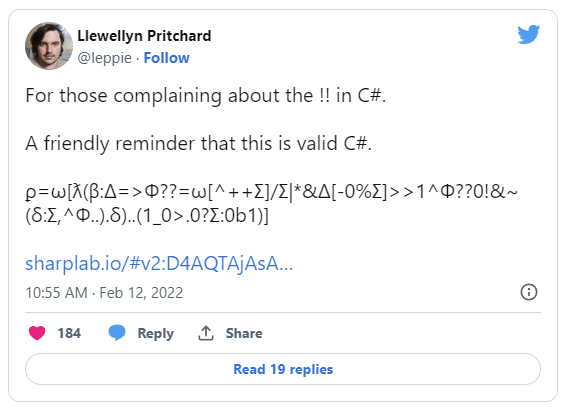
Excuse me? I'm extremely happy this came with a Sharplab snippet. Let's break it down. First, looking at the full original code:
using System;
unsafe class Program
{
delegate void bar(int* i);
static Index ƛ(bar β) => default;
static void Main(string[] args)
{
int[] ω = { };
int Ʃ = 42;
int? Φ = 10;
var ϼ = ω;
ϼ=ω[ƛ(β:Δ=>Φ??=ω[^++Ʃ]/Ʃ|*&Δ[-0%Ʃ]>>1^Φ??0!&~(δ:Ʃ,^Φ..).δ)..(1_0>.0?Ʃ:0b1)];
}
}
Then after we make some changes we can begin to more easily pull the example apart:
unsafe class Program
{
delegate void DelegatePointer(int* i);
static Index Indexer(DelegatePointer delegatePointer) => default;
static void Main(string[] args)
{
int[] intArray = { };
int position = 42;
int? nullableValue = 10;
var intArray2 = intArray;
intArray2 = intArray[Indexer(
delegatePointer: x => nullableValue ??= intArray[^++position] / position | *&x[-0 % position] >> 1 ^ nullableValue ?? 0! & ~(δ: position, ^nullableValue..).δ)..(1_0 > .0 ? position : 0b1)];
}
}
Where we have:
- A delegate that takes in a pointer
- The newish
Index
type, introduced to help with Ranges. - A whole bunch of arrays and
int
types
Then we get into the big complex line, which is made up of:
- Array indexing
- The
Index
from earlier - Lambda expressions
- A lot of pointer operators
- And some ranges thrown in
So it's still hard to read, but unobscured by Greek and broken down to the sum of its parts it's a little more manageable and no less fun to show off to others!
Not as Much Greek
Found this in my feed:
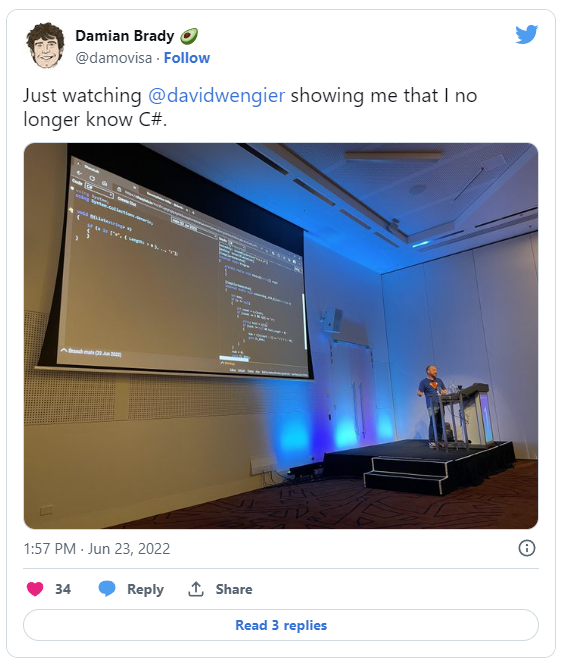
It's a less Greek version of what we just saw. If you zoom in, you can see it's also made up of patterns, and the new range work.
Tolstoy in C#
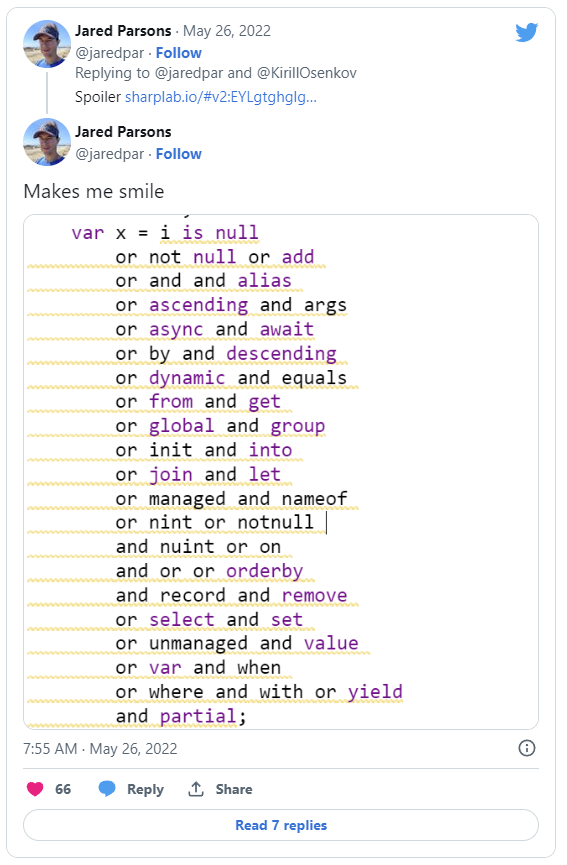
There's a lot more natural language in C# when compared to lower level languages, or even C# of a decade ago. Since C# 7 we've had Patterns which have been iterated on since meaning we can get to the fun state above.
Sure, there's a little cheating involved as they're not all keywords (check the Sharplab link in the tweet) but it's still fun to let it sink in after seeing it for the first time.
Structs Have a Fluid Identity
Being bored as a nerd, you're bound to be the stereotype at least once, and I went looking for interesting C# snippets and found that Struct
s can reassign their this
value.
Full credit to Omer Mor for this StackOverflow answer:
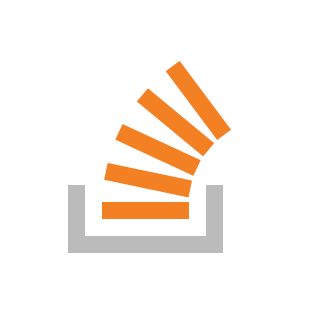
Maybe there's a cool reason why, but it feels.. weird:
public struct Teaser
{
public void Foo()
{
this = new Teaser();
}
}
There's Never Enough Null Checks
This tweet right here:
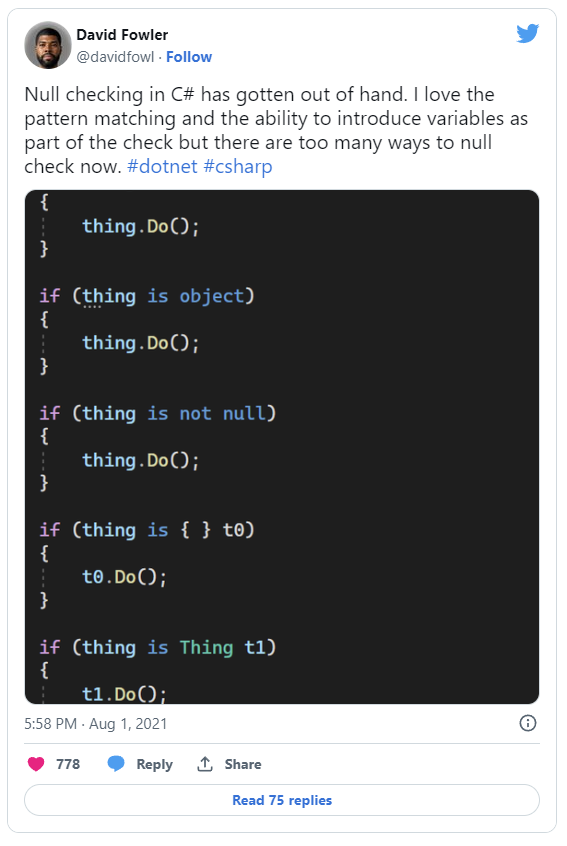
Shows a wild amount of null
checks. Whether you believe C# has too many ways to do the same thing or not, this amount is impressive. Though if you frame it so, you can have this as an advantage of C#, where your niche requirement is met by some obscure and valid operation.
In fact, this tweet pushed me to keep a collection of other interesting C# snippets, including the null
checks above:
I did run across this meme that I feel has some truth for the future:
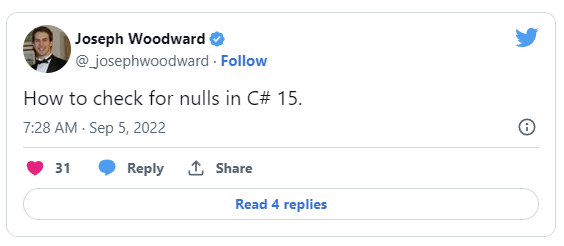
What the Compiler Doin'?
Maybe in the future I'll do a full write up exploring these but if you haven't seen, Bartosz Adamczewski does really fascinating writeups and showcasing of compiler manipulations in .NET. You can view all of them on his website or check out a few of his tweets here:
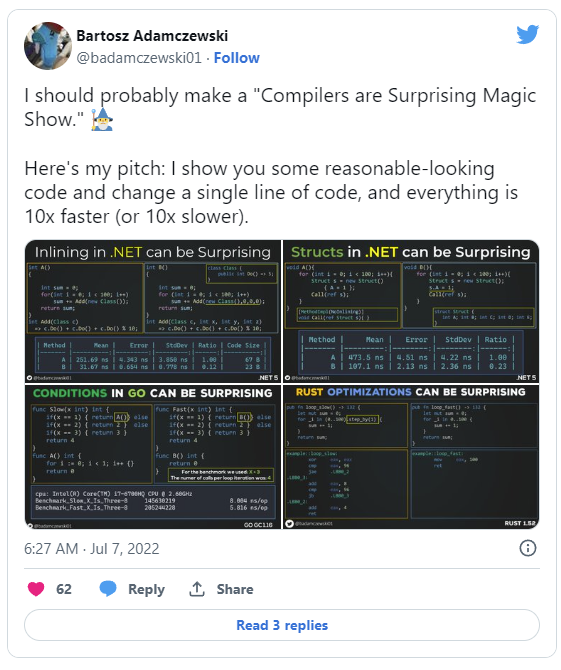
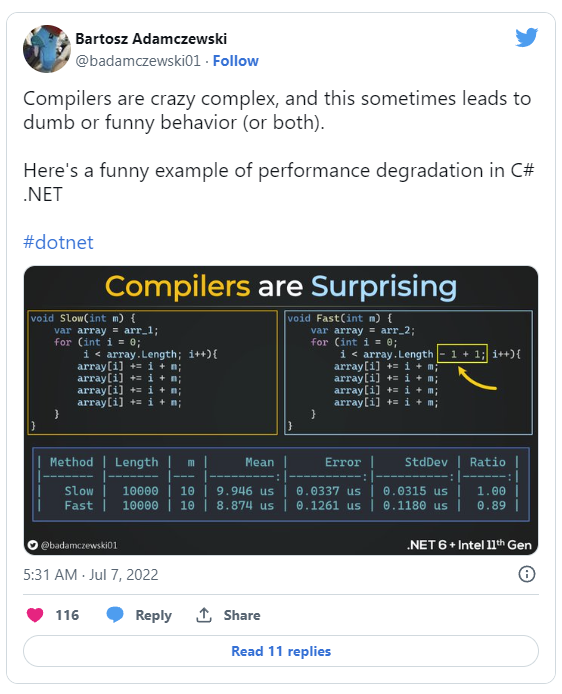
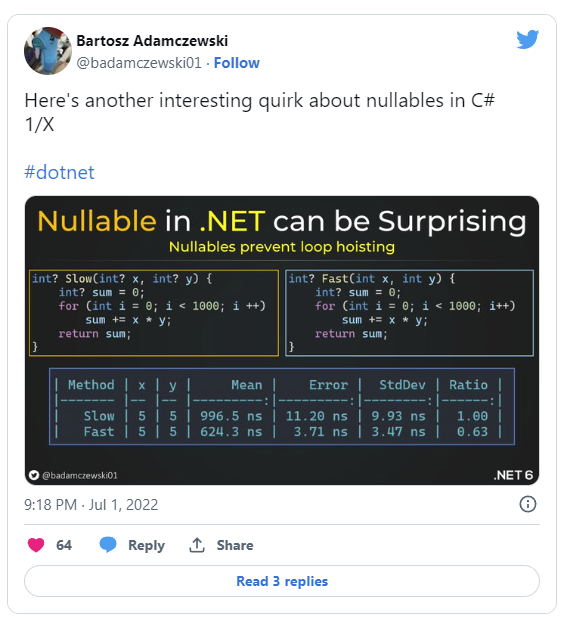
To Conclude
Since getting deeper into the .NET community in 2022, I've been collecting little snippets of oddity and fun. I'm sure there's a lot more out there and maybe in the future I'll collect enough to write up another post. I hope you found this as enjoyable as I do!