What Does Asterisk *, Plus +, and Double Colon :: Mean in ASP.NET Core URLs?
Sometimes in a .NET project you might see a wildcard in the URL like http://*:5400 or perhaps two colons in the console output like http://[::]:5400. This post will explain what they are, and how they work.
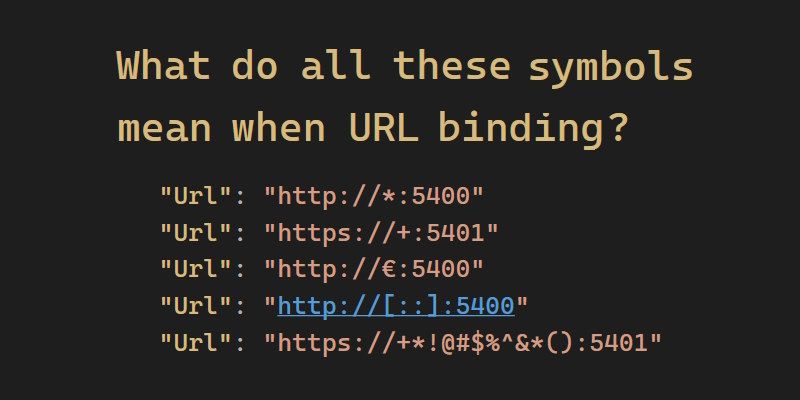
I saw https://+:5401
in a project and assumed the plus sign was a wildcard. Then in another project in the same solution I saw https://*:5402
with a asterisk - confused I wondered what the difference was. This post is my discovery with full references and links.
What Is URL Binding?
Your web application will need to attach itself to a URL to listen to for taking requests and URL binding is how we do it. It's why, for example, you can hit http://locahost:5000
and access your web application.
If you're looking for all the different ways you can do URL binding, check out 5 ways to set the URLs for an ASP.NET Core app by Andrew Lock for a fantastic and easily accessible list of ways to bind.
Before we head into answering the question of this post, we need to look at the differences between launchSettings.json
and appsettings.json
because this is important to understanding a key concept of this question - where wildcards can be used.
launchSettings.json
Configuration only used for the local development machine. This can have all sorts of information that is useful in development environments including different "profiles" to create setup scenarios for your development purposes. Important here is that the http and https profiles below will allow us to host via the Kestrel web server rather than something else like IIS Express.
Let's look at the default when creating a basic .NET minimal API project:
"profiles": {
"http": {
"commandName": "Project",
"dotnetRunMessages": true,
"launchBrowser": true,
"applicationUrl": "http://localhost:5093",
"environmentVariables": {
"ASPNETCORE_ENVIRONMENT": "Development"
}
},
"https": {
"commandName": "Project",
"dotnetRunMessages": true,
"launchBrowser": true,
"applicationUrl": "https://localhost:7192;http://localhost:5093",
"environmentVariables": {
"ASPNETCORE_ENVIRONMENT": "Development"
}
}
}
appsettings.json
Application configuration file, more closely related to the non-development application runtime.
Let's take a look at a simple Kestrel configuration:
"Kestrel": {
"Endpoints": {
"Http": {
"Url": "http://localhost:5400"
},
"Https": {
"Url": "https://localhost:5401"
}
}
}
If you have URL configuration in both launchSettings.json and appsettings.json, your console output will warn you about it and take the appsettings.json values:

Now that we understand this, let's answer the questions.
Wildcard Binding
First up, we can read the documentation around URL Prefixes, which states:
Host names,*
, and+
, aren't special. Anything not recognized as a valid IP address orlocalhost
binds to all IPv4 and IPv6 IPs.
And for the sake of the examples below, HTTP vs HTTPS does not matter, what you see in this post will work for either.
Let's look at launchSettings.json first. If we replace localhost
with *
, Visual Studio will not run the application:
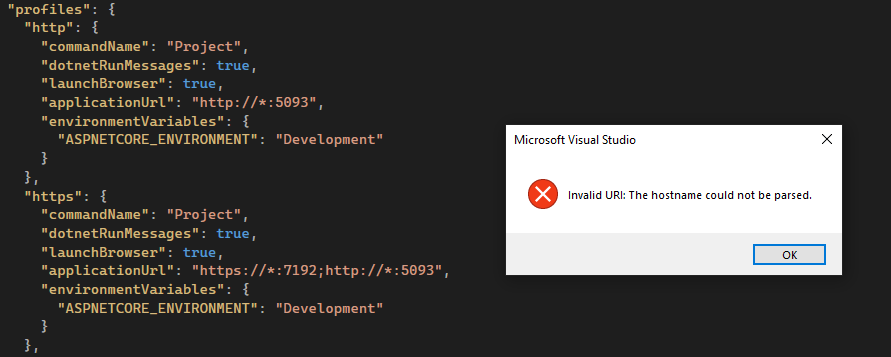
*
in the URLs.However, if we do this in appsettings.json where our Kestrel configuration lives:
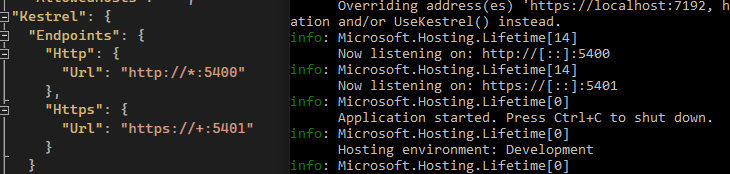
"Kestrel": {
"Endpoints": {
"Http": {
"Url": "http://*:5400"
},
"Https": {
"Url": "https://+:5401"
}
}
}
Looking at this post by Joseph Woodward you can put nearly any symbol ("Anything not recognized as a valid IP address") there instead:
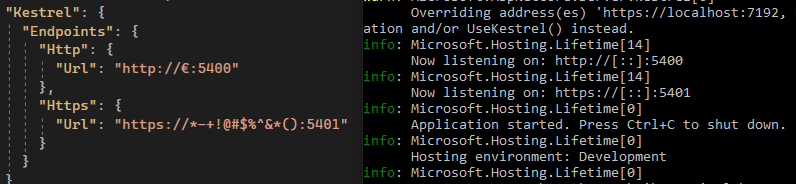
"Kestrel": {
"Endpoints": {
"Http": {
"Url": "http://€:5400"
},
"Https": {
"Url": "https://*-+!@#$%^&*():5401"
}
}
}
So to answer the first couple of questions: With appsettings.json we can configure Kestrel with wildcards such as *
or +
to bind to all IPv4 and IPv6 IPs. Meaning we can use a whole range of symbols instead to do it. However, we cannot do this with URLs in launchSettings.json.
IPV6 Binding
This part answers what a double colon ::
means. You've seen it already in the console output above when binding to all IP addresses:
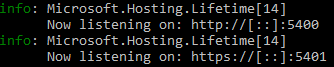
Following the same documentation as before, we can get some special cases:
0.0.0.0
is a special case that binds to all IPv4 addresses[::]
is the IPv6 equivalent of IPv40.0.0.0
- our answer!
Let's look at what happens with each, starting with 0.0.0.0
:
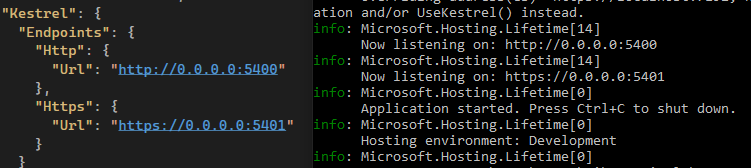
0.0.0.0
showing up as 0.0.0.0
in the console."Kestrel": {
"Endpoints": {
"Http": {
"Url": "http://0.0.0.0:5400"
},
"Https": {
"Url": "https://0.0.0.0:5401"
}
}
}
Then with [::]
:
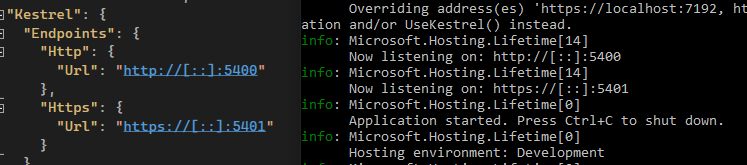
[::]
showing up as [::]
in the console."Kestrel": {
"Endpoints": {
"Http": {
"Url": "http://[::]:5400"
},
"Https": {
"Url": "https://[::]:5401"
}
}
}
Notice how configuring the URL as [::]
shows up the same as the wildcards we were looking at earlier. We can even compare them:
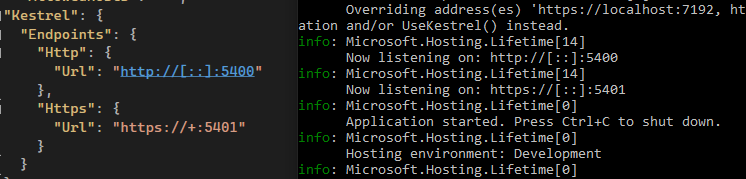
[::]
and +
for binding."Kestrel": {
"Endpoints": {
"Http": {
"Url": "http://[::]:5400"
},
"Https": {
"Url": "https://+:5401"
}
}
}
To Conclude
Asterisks *
and plus +
when used are wildcards that will bind to to everything and the double colon [::]
looks to be another notation of this, but specifically derived from the IPV6 world.
I have an example repo as an easy way to play with all this:
Other References
References not linked in the post so far:
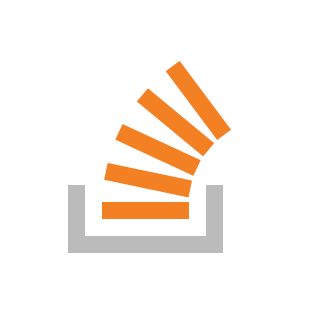

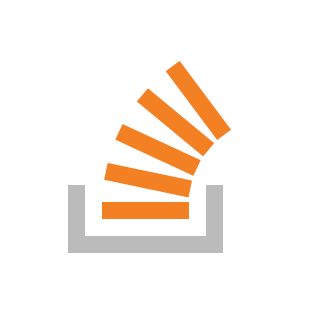